210204_TIL
🎄오늘 한 일
✔️ 1. ChatterBox 웹 앱 만들기
어제부터 진행하였던 chatter Box를 성공적으로 완성하였다.
원하는 기수를 선택하고, 이름과 메세지를 적고 Post 버튼을 누르면
서버로 해당 메세지를 전송하고 생성하게 된다.
이후 바로 다시 서버에서 모든 메세지를 가져와 기존 돔을 비우고 다시 렌더링하여 메세지가 추가되는 것까지 구현하였다.
추가로 server clear 버튼과 DOM clear 버튼을 만들어서
각각 서버에 있는 모든 메시지를 삭제하는 역할, DOM에 모든 메세지를 지우는 역할을 하도록 구현하였다.
🎄chatterbox
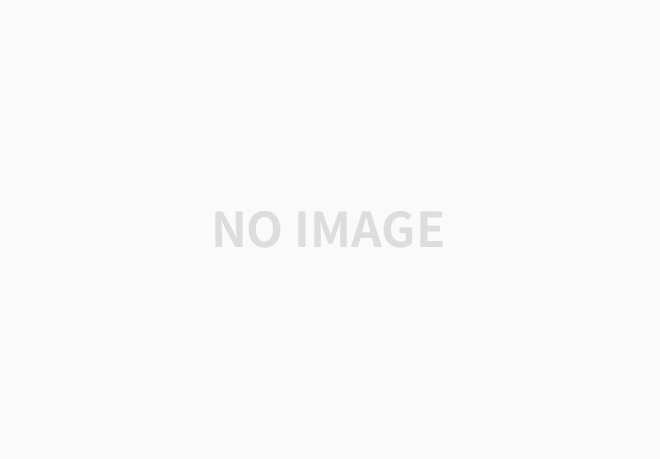
🎄코드 분석 및 개선
코드 분석 (함수단위)
1. init
-
처음 앱 시작시 실행되는 코드로
메시지를 전부 가져와 화면에 뿌려주고, 버튼들에 이벤트를 연결해주는 기능 (추가)
//before
init: () => {
app.renderAllMessages();
}
//After *이벤트 연결함수 추가
init: () => {
app.addEventHandler();
app.renderAllMessages();
},
2. fetch
- 서버에 fetch API로 get요청을 하여 메세지를 받아오는 함수
//before *fetch()로 resp을 받아와서 callback을 실행시켜준다.
fetch: (callback) => {
return window.fetch(app.server)
.then(callback)
}
//After *모든 fetch함수는 resp이 아닌 json()으로 바꾼 데이터를 사용하므로,
// json데이터를 리턴하여 callback함수를 이어 실행하도록 수정
fetch: (callback) => {
return window.fetch(app.server)
.then(resp => resp.json())
.then(callback)
},
3. send
- 서버에 fetch API로 POST요청을 하여 메세지를 새로 생성하는 함수
//before * message를 받아 fetch API를 통해 POST 요청한다.
send: (message) => {
window.fetch(app.server, {
method: 'POST',
body: JSON.stringify(message),
headers: {
'Content-Type': 'application/json'
}
})
}
//After * 메세지 등록이후 후속작업을 위한 callback 실행부분을 추가
send: (message, callback) => {
window.fetch(app.server, {
method: 'POST',
body: JSON.stringify(message),
headers: {
'Content-Type': 'application/json'
}
})
.then(resp => resp.json())
.then(callback)
},
4.clearMessages
- DOM에 있는 모든 chat Element를 삭제하는 함수
// 채팅이 들어가는 엘리먼트의 자식이 존재한다면 반복하여 모두 지우는 것으로 구현
clearMessages: () => {
let chatsElement = document.querySelector('#chats');
while (chatsElement.hasChildNodes()) {
chatsElement.removeChild(chatsElement.firstChild)
}
console.log("돔의 메세지를 전부 지웠습니다")
},
5.renderMessage
- 하나의 message를 받아서 chatElment를 만들고 값을 채워 채팅메세지를 렌더링 하는 함수
// 하나의 message 데이터를 받아, chat elemnent를 만들어 데이터를 연결시켜주고, 렌더링한다.
renderMessage: (data) => {
const chatsElement = document.querySelector('#chats');
const chatElement = document.createElement('div');
chatElement.classList.add('chat');
const userNameElement = document.createElement('div');
userNameElement.classList.add('username')
const messageSpan = document.createElement('span');
messageSpan.textContent = data.text;
userNameElement.textContent = `${data.roomname}: ${data.username}`;
chatElement.appendChild(userNameElement);
chatElement.appendChild(messageSpan);
chatsElement.appendChild(chatElement);
},
6.renderAllMessages
- 서버에 fetch API로 get요청을 하여 모든 메세지를 받아와서, 전부 렌더해주는 함수
// 받아온 데이터를 순회하며 renderMessage( )를 실행한다.
renderAllMessages: () => {
app.fetch(data => {
data.forEach(el => app.renderMessage(el))
});
console.log("메세지를 전부 가져왔습니다")
}
7.onSubmit
- post 버튼 클릭 시 실행되는 함수 : 서버에 메세지 보내고, DOM을 지운 후, 다시 데이터를 받아와서 렌더링 하는 순서로 이루어짐
//before *app.fetch() 와 app.renderAllMessages() 안에서 app.fetch() 두번 실행되는 문제
onSubmit: () => {
const userInput = document.querySelector('.inputUser')
const chatInput = document.querySelector('.inputChat')
const room = document.querySelector('.roomSelect')
let message = {
username: userInput.value,
text: chatInput.value,
roomname: room.value
}
console.log('제출되었습니다')
app.send(message)
app.clearMessages()
app.fetch();
app.renderAllMessages();
},
//After *app.send() 이후 후속작업으로 실행될 수 있도록 callback으로 넣어주었다
// + app.fetch() 중복실행제거
onSubmit: () => {
...
console.log('제출되었습니다')
app.send(message, () => {
app.clearMessages()
app.renderAllMessages();
})
},
8.onServerClear
- 서버에서 API 문서 중 모든 서버데이터를 지우는 요청을 날린다.
onServerClear: () => {
window.fetch(app.clearServer, {
method: 'POST',
})
console.log("서버의 메세지를 전부지웠습니다")
},
9.addEventHandlers
- 함수 첫 실행시 버튼들을 dom에서 가져와 각각 맞는 이벤트핸들러 함수를 연결시켜주는 기능
//before *app.init() 아래 따로 존재
app.init();
const postBtn = document.querySelector('.postBtn');
const clearBtn = document.querySelector('.domClearBtn');
const serverClearBtn = document.querySelector('.serverClearBtn')
if (postBtn) {
postBtn.addEventListener("click", app.onSubmit)
}
if (clearBtn) {
clearBtn.addEventListener("click", app.clearMessages)
}
if (serverClearBtn) {
serverClearBtn.addEventListener("click", app.onServerClear)
}
// After *함수로 묶어서 init안에서 바로 실행되도록 수정
init: () => {
app.addEventHandler();
app.renderAllMessages();
},
addEventHandler: () => {
const postBtn = document.querySelector('.postBtn');
...
}
마무리 Think:
오늘 live server 라는 vscode Extension을 통해 첫 온라인 집들이를 해보았다.
공동개발도구가 이렇게 재미있는 하나의 놀이가 될 줄은 몰랐다.. ㅎㅎㅎ
일이 힘들수록 사소한 즐거움이 크게 느껴지는 법인데, 딱 그런느낌이 들었다. 하하호홓
init: () => { app.addEventHandler(); app.renderAllMessages(); }, addEventHandler: () => { const postBtn = document.querySelector('.postBtn'); const clearBtn = document.querySelector('.domClearBtn'); const serverClearBtn = document.querySelector('.serverClearBtn') if (postBtn) { postBtn.addEventListener("click", app.onSubmit) } if (clearBtn) { clearBtn.addEventListener("click", app.clearMessages) } if (serverClearBtn) { serverClearBtn.addEventListener("click", app.onServerClear) } }
: )